Deploy Next.js with PM2
You can deploy Next.js with PM2 to keep your application running in the background. PM2 is a process manager for Node.js applications that will help you manage your Next.js application.
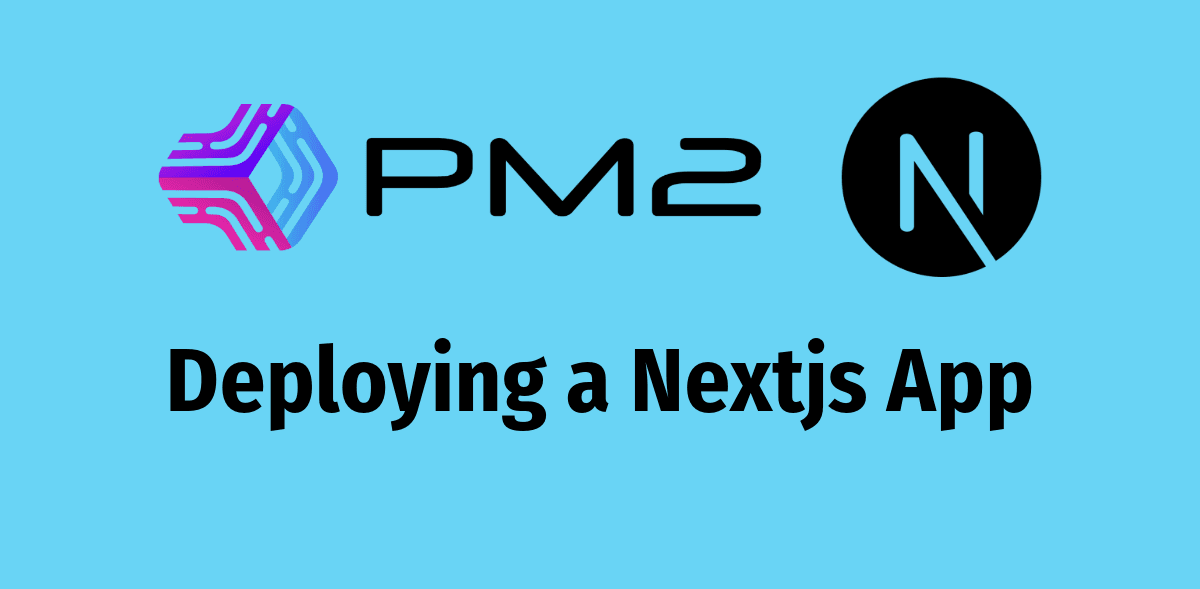
Introduction
Next.js is a popular React framework that allows you to build server-side rendered applications with ease. It provides a great developer experience and is used by many companies to build their websites.
PM2 is a process manager for Node.js applications that will help you manage your Next.js application. It allows you to keep your application running in the background and provides features like automatic restarts, logging, and monitoring.
Prerequisites
To complete this tutorial, you will need access to an Ubuntu server as a regular, non-root sudo user, and a firewall enabled on your server.
Step 1 — Installing Nginx
If this is your first time setting up a server. you will need to update your package list and install Nginx.
sudo apt update
sudo apt install nginx
Once the installation is complete, we will need to open port 22 for SSH and port 80 for HTTP traffic.
sudo ufw allow OpenSSH
sudo ufw allow 'Nginx Full'
sudo ufw enable
You can check the status of the firewall by running:
sudo ufw status
Step 2 — Installing Node.js
We will use NVM to install Node.js. NVM is a version manager for Node.js that allows you to install multiple versions of Node.js on your system.
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
To start using NVM, you will need to close and reopen your terminal or run the following command:
source ~/.bashrc
Now you can install Node.js by running:
npm install --lts
Step 3 — Installing PM2
PM2 is a process manager for Node.js applications that will help you manage your Next.js application. You can install PM2 globally by running:
npm install pm2 -g
Step 4 — Setting up your Next.js application
To deploy your Next.js application with PM2, you will need to create an ecosystem file. This file will contain the configuration for your Next.js application.
Create a new file called ecosystem.config.js
in the root of your Next.js application and add the following configuration:
module.exports = {
apps: [
{
name: 'nextjs-app',
script: './node_modules/.bin/next',
args: 'start',
env: {
PORT: 3000,
NODE_ENV: 'production',
},
},
],
}
This configuration will start your Next.js application in production mode on port 3000.
Step 5 — Configure nginx to proxy requests to your Next.js application
To configure Nginx to proxy requests to your Next.js application, you will need to create a new server block in your Nginx configuration.
Create a new file called nextjs
in the /etc/nginx/sites-available
directory and add the following configuration:
server {
listen 80;
server_name your_domain.com;
location / {
proxy_pass http://localhost:3000;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
Once you have created the configuration file, you will need to enable the server block by creating a symbolic link to the sites-enabled
directory:
sudo ln -s /etc/nginx/sites-available/nextjs /etc/nginx/sites-enabled/
You can test your Nginx configuration by running:
sudo nginx -t
If the configuration is correct, you can restart Nginx by running:
sudo systemctl restart nginx
Step 6 — Starting your Next.js application with PM2
To start your Next.js application with PM2, you will need to run the following command in the root of your Next.js application:
pm2 start ecosystem.config.js
This will start your Next.js application in the background and keep it running even after you close your terminal.
You can check the status of your application by running:
pm2 status
Step 7 — Make your Next.js application start on boot
To make your Next.js application start on boot, you will need to generate a startup script for PM2.
You can generate a startup script by running:
pm2 startup
This will output a command that you can run to set up PM2 to start on boot.
Step 8 — Install SSL certificate (Optional)
If you want to secure your Next.js application with SSL, you can install a free SSL certificate from Let's Encrypt.
You can install Certbot by running:
sudo apt install certbot python3-certbot-nginx
Once Certbot is installed, you can request a new SSL certificate by running:
sudo certbot --nginx
Follow the on-screen instructions to install the SSL certificate.
Conclusion
In this tutorial, you learned how to deploy your Next.js application with PM2. You can now keep your application running in the background and manage it with PM2. You also learned how to configure Nginx to proxy requests to your Next.js application and how to secure your application with SSL.